
PHP Recursive copy/delete functions
In a recent project I needed quite often to delete or copy whole directories. And since PHP doesn’t seem to have such – I created these:
Remove Directory
protected function rmdir($path) : void
{
if (is_dir($path)) {
$children = scandir($path);
foreach ($children as $child) {
if ('.' === $child || '..' === $child) {
continue;
}
$local_path = "{$path}/{$child}";
if ("dir" === filetype($local_path)) {
self::rmdir($local_path);
} else {
unlink($local_path);
}
}
rmdir($path);
}
}
Copy Directory
protected function cpdir($src, $dest) : void
{
if (!is_dir($dest)) {
mkdir($dest);
}
if (is_dir($src)) {
$children = scandir($src);
foreach ($children as $child) {
if (in_array($child, ['.', '..'])) {
continue;
}
$source = "{$src}/{$child}";
$destination = "{$dest}/{$child}";
if (is_dir($source)) {
self::cpdir($source, $destination);
} else {
copy($source, $destination);
}
}
}
}
Similar blog posts
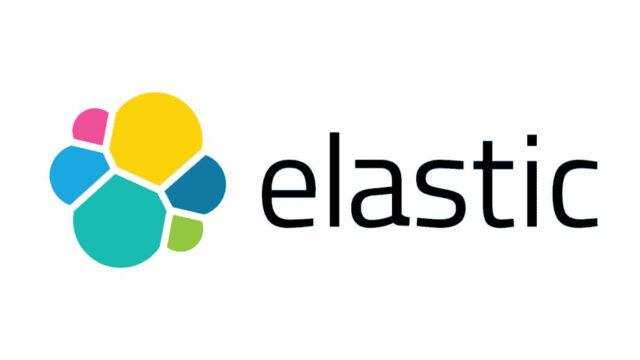
Elasticsearch cheat sheet
Search Count AND query OR query Query with date range Multiple Queries Or a query inside a query Sort by a date containing field Query with regex Point In Time...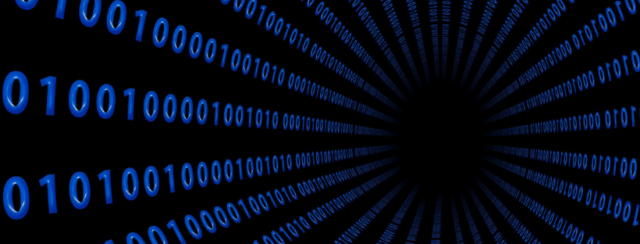
Odroid Sensors
Not long ago I bought an Odroid ARM based mini PC, and among many issues, turned out that the system uses some rather strange scheme for controlling the fan speed....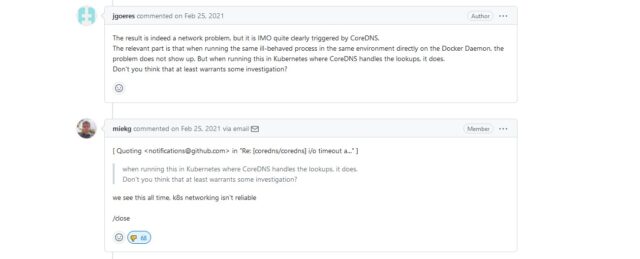
“Kubernetes network isn’t reliable”
Recently, while trying to figure out an extrelemly annoying problem I was having with Kubernetes – I stumbled upon this marvel of a github issue. So if you ever need...